Dusty's Pi-Rizz Display
CPU: 75%
Temp: 68°F
Mem: 45%
Username
12:34:56
Jan 24, 2025
Wednesday
(^_^)
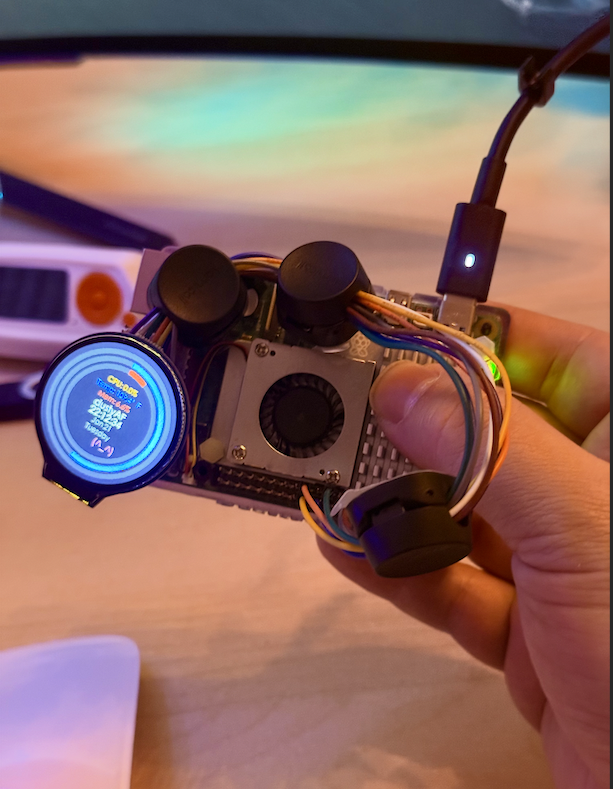
Project Overview
The Pi-Rizz Display is a stylish system monitor built using a Raspberry Pi and a circular LCD display. It shows real-time system statistics such as CPU usage, temperature, memory usage, time, and date in a visually appealing format inspired by Apple's design aesthetic.
Materials Needed
- Raspberry Pi 5 (or compatible model)
- Waveshare 1.28inch Touch LCD
- MicroSD card with Raspberry Pi OS installed
- Python 3
- Optional: A font file like Font00.ttf for a custom look
Pro Tip
The Raspberry Pi 5 is the perfect wingman for Dusty's Pi-Rizz Display. Its beefed-up performance ensures smooth animations and real-time data updates that'll make your display irresistible. Plus, its improved thermal management keeps things cool under pressure - essential for maintaining that effortless Pi-Rizz.
Setup Steps
- Prepare Your Raspberry Pi
- Install Raspberry Pi OS on your MicroSD card
- Enable SPI and I2C interfaces using raspi-config
- Install Necessary Software
sudo apt update sudo apt upgrade -y sudo apt install python3-pip -y sudo pip3 install psutil Pillow
- Connect the LCD
Follow the wiring diagram provided by Waveshare for your specific LCD model.
Detailed wiring diagram showing pin connections between Raspberry Pi and the Waveshare 1.28inch LCD display. Color legend: VCC (red), GND (black), DIN (blue), CLK (yellow), CS (orange), DC (green), RST (gray), BL (purple)
- Get the Code
git clone https://github.com/waveshare/e-Paper.git cd e-Paper/RaspberryPi_JetsonNano/python/lib sudo python3 setup.py install
- Write the Python Script
Create a file named display_info.py with the following code:
#!/usr/bin/python # -*- coding: UTF-8 -*- import os import sys import time import logging import spidev as SPI sys.path.append("..") from lib import LCD_1inch28, Touch_1inch28 from PIL import Image, ImageDraw, ImageFont import psutil import math import random from datetime import datetime # Raspberry Pi pin configuration: RST = 27 DC = 25 BL = 18 # Setup logging logging.basicConfig(level=logging.DEBUG) def draw_ring_with_silhouette(draw, x, y, radius, thickness, percentage, color, silhouette_color): # Draw silhouette for full ring draw.arc((x - radius, y - radius, x + radius, y + radius), 0, 360, fill=silhouette_color, width=thickness) # Draw actual filled part with rounded corners start_angle = -math.pi / 2 # Start from the top end_angle = start_angle + 2 * math.pi * percentage / 100 for i in range(thickness): if i == 0 or i == thickness - 1: # First and last lines for rounded corners draw.arc((x - radius + i, y - radius + i, x + radius - i, y + radius - i), start_angle * 180 / math.pi, end_angle * 180 / math.pi, fill=color, width=1) else: draw.arc((x - radius + i, y - radius + i, x + radius - i, y + radius - i), start_angle * 180 / math.pi, end_angle * 180 / math.pi, fill=color, width=1) def draw_emoticon(draw, x, y, emoticon, font, color): draw.text((x, y), emoticon, font=font, fill=color) # Animation variables ring_animation = 0 try: # Initialize display disp = LCD_1inch28.LCD_1inch28() disp.Init() disp.clear() # Create blank image for drawing in dark mode. image = Image.new("RGB", (disp.width, disp.height), "#1C1C1E") # Dark background for dark mode draw = ImageDraw.Draw(image) # Font setup with Font00.ttf title_font = ImageFont.truetype("../Font/Font00.ttf", 16) info_font = ImageFont.truetype("../Font/Font00.ttf", 12) emoticon_font = ImageFont.truetype("../Font/Font00.ttf", 16) # Assuming Font00 supports these characters # Color scheme for dark mode - light text on dark background title_color = "#F5F5F7" # Light grey for titles cpu_color = "#FF9500" # Warm orange for CPU temp_color = "#007AFF" # Blue for temperature mem_color = "#FF3B30" # Red for memory silhouette_color = "#323232" # Dark grey for silhouettes emoticon_color = "#FF69B4" # Pink for the emoticon info_color = "#8E8E93" # Mid grey for information # Pi-Rizz emoticons pi_rizz_emoticons = [ "(͠≖ ͜ʖ͠≖)", # Cool "(ᵔᴥᵔ)", # Happy "(¬‿¬)", # Smug "(╯°□°)╯", # Excited "(ಠ_ಠ)", # Disapproval (high CPU load) ] while True: draw.rectangle((0, 0, disp.width, disp.height), fill="#1C1C1E") # Refresh with dark mode color # CPU Usage, Temperature, and Memory Usage try: cpu_percent = psutil.cpu_percent(interval=0.5) # Short interval for smoother animation temp_c = psutil.sensors_temperatures()['cpu_thermal'][0].current if 'cpu_thermal' in psutil.sensors_temperatures() else 0 temp_f = (temp_c * 9/5) + 32 # Convert to Fahrenheit temp_percent = min((temp_c / 80) * 100, 100) # Assuming 80°C is max temp for visual mem_percent = psutil.virtual_memory().percent except KeyError: logging.warning("CPU temperature sensor not found. Pi-Rizz levels may be affected.") temp_f, temp_percent = 0, 0 # Fallback values center_x, center_y = disp.width // 2, disp.height // 2 max_radius = min(disp.width, disp.height) // 2 - 10 # Leave a bit of margin # Animation for rings ring_animation = (ring_animation + 5) % 360 # Draw CPU Usage Ring (outermost) draw_ring_with_silhouette(draw, center_x, center_y, max_radius, 8, cpu_percent, cpu_color, silhouette_color) # Draw Temperature Ring (middle) draw_ring_with_silhouette(draw, center_x, center_y, max_radius - 13, 8, temp_percent, temp_color, silhouette_color) # Draw Memory Usage Ring (innermost) inner_radius = max_radius - 26 draw_ring_with_silhouette(draw, center_x, center_y, inner_radius, 8, mem_percent, mem_color, silhouette_color) # Text inside the innermost ring with matching colors text_y_offset = -54 # Start above center, adjust as needed line_spacing = 15 draw.text((center_x, center_y + text_y_offset), f"CPU: {cpu_percent:.1f}%", font=info_font, fill=cpu_color, anchor="mm") text_y_offset += line_spacing draw.text((center_x, center_y + text_y_offset), f"Temp: {temp_f:.1f}°F", font=info_font, fill=temp_color, anchor="mm") text_y_offset += line_spacing draw.text((center_x, center_y + text_y_offset), f"Mem: {mem_percent:.1f}%", font=info_font, fill=mem_color, anchor="mm") text_y_offset += line_spacing draw.text((center_x, center_y + text_y_offset), "dustyAF", font=title_font, fill=title_color, anchor="mm") text_y_offset += line_spacing current_time = datetime.now().strftime('%H:%M:%S') draw.text((center_x, center_y + text_y_offset), current_time, font=title_font, fill=title_color, anchor="mm") text_y_offset += line_spacing # Date and Day of the Week - now using info_font for consistency date_text = datetime.now().strftime('%b %d') day_text = datetime.now().strftime('%A') draw.text((center_x, center_y + text_y_offset), date_text, font=info_font, fill=info_color, anchor="mm") text_y_offset += line_spacing draw.text((center_x, center_y + text_y_offset), day_text, font=info_font, fill=info_color, anchor="mm") # Pi-Rizz Emoticon - now static within the inner rings if cpu_percent < 30: emoticon = pi_rizz_emoticons[1] # Happy elif 30 <= cpu_percent < 50: emoticon = pi_rizz_emoticons[0] # Cool elif 50 <= cpu_percent < 70: emoticon = pi_rizz_emoticons[2] # Smug elif 70 <= cpu_percent < 90: emoticon = pi_rizz_emoticons[3] # Excited else: emoticon = pi_rizz_emoticons[4] # Disapproval # Position the emoticon just above the bottom text inside the rings emoticon_y = center_y + inner_radius - 43 # Adjust this value to place emoticon appropriately draw_emoticon(draw, center_x, emoticon_y, emoticon, emoticon_font, emoticon_color) disp.ShowImage(image) time.sleep(0.1) # Faster update for smoother animation except IOError as e: logging.error(f"An error occurred with the Pi-Rizz display: {e}") except KeyboardInterrupt: logging.info("Pi-Rizz display interrupted by user, exiting with style...") disp.module_exit() sys.exit()
- Set Up to Run at Boot
Create a systemd service file to run your script at startup:
sudo nano /etc/systemd/system/display_info.service
Add the following content, adjusting paths as necessary:
[Unit] Description=Display System Information on LCD After=network.target [Service] ExecStart=/usr/bin/python3 /home/your_username/project/display_info.py WorkingDirectory=/home/your_username/project StandardOutput=inherit StandardError=inherit Restart=always User=your_username Group=your_username [Install] WantedBy=multi-user.target
Enable and start the service:
sudo systemctl daemon-reload sudo systemctl enable display_info.service sudo systemctl start display_info.service
- Check and Troubleshoot
- Check service status:
sudo systemctl status display_info.service
- View logs:
sudo journalctl -u display_info.service
- Troubleshoot permissions: Ensure your user has read/write access to the necessary files and GPIO.
- Check service status:
Customization Tips
- Replace Font00.ttf with another TTF file for different typography
- Add network status or disk space monitoring
- Customize ring colors and animations
- Add weather data integration
Performance Tips
- Use hardware acceleration when possible
- Optimize refresh rates for smooth updates
- Implement double buffering for animations
- Cache frequently used graphics
Final Notes on Maximizing Your Pi-Rizz
- 🔬 Experiment with different animations to keep your Pi-Rizz fresh and eye-catching
- 🛠️ Add touch gestures for interactive features that'll really impress onlookers
- 🔒 Keep your Pi-Rizz secure by following best practices when running with elevated privileges
- 🌐 Show off your Pi-Rizz in the Raspberry Pi community and inspire others with your modifications